Light weight and fast image load jQuery to get best user experience
Nov 03, 24 Devgod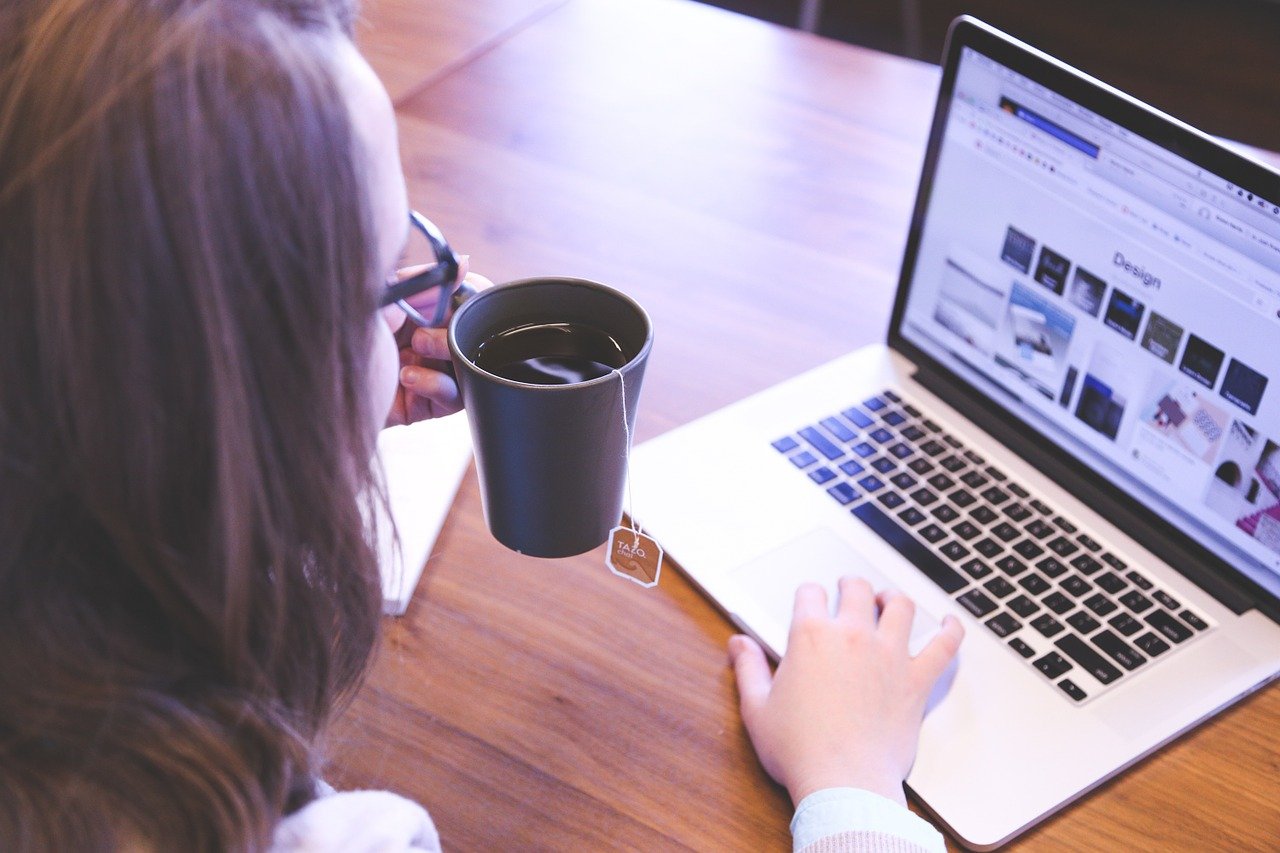
In the world of web development, a seamless and fast image loading experience is essential to keep visitors engaged. High-resolution images can slow down websites, impacting user experience and even SEO rankings. Fortunately, using jQuery along with lazy-loading techniques can help optimize image loading by loading images only when they appear on the screen, reducing load times and enhancing user experience.
In this guide, we’ll explore the use of jQuery for lightweight image loading and integrate a popular library, jquery.lazy. This library is well-suited for optimizing image loads and can be easily implemented in any website.
Step 1: Setting Up jQuery and Lazy-Loading Library
To start, include the jQuery library and the jquery.lazy plugin in your HTML document. You can add these libraries through a CDN (Content Delivery Network), which will speed up the loading process.
Add the following code within the <head>
tag of your HTML:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery.lazy/1.7.11/jquery.lazy.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery.lazy/1.7.11/jquery.lazy.plugins.min.js"></script>
Step 2: Writing HTML Structure for Lazy-Loaded Images
Instead of loading all images at once, we’ll set up images to load only when they are scrolled into view. This approach saves bandwidth and reduces initial loading time, making the page more responsive.
Use a data-src
attribute in place of the standard src
attribute. This way, images won’t load until triggered by jQuery.
<div class="image-container"> <img class="lazy" data-src="image1.jpg" alt="Image 1" width="600" height="400"> <img class="lazy" data-src="image2.jpg" alt="Image 2" width="600" height="400"> <img class="lazy" data-src="image3.jpg" alt="Image 3" width="600" height="400"> </div>
Step 3: Implementing the Lazy Loading Logic
Using jQuery, we can now initialize lazy loading on images with the data-src
attribute. The jquery.lazy plugin allows for a customizable lazy-loading experience, including fade-in effects and adjustable loading thresholds.
Add the following script at the end of the body tag:
<script> $(function() { $('.lazy').Lazy({ effect: "fadeIn", effectTime: 500, threshold: 0 }); }); </script>
Additional Benefits of jQuery Lazy Loading
- Improves Page Load Speed: By deferring off-screen images, the initial page load is faster.
- Saves Bandwidth: Only loads images when needed, saving data, especially on mobile devices.
- Enhances User Experience: With a more responsive website, users will have a smoother, lag-free experience.
- SEO Benefits: Google considers page load speed in its rankings, so lazy loading can help improve your SEO.
Customizing Lazy Load Settings
You can further customize how and when images load based on your site’s needs. Here’s an example with additional settings:
<script> $(function() { $('.lazy').Lazy({ effect: "fadeIn", effectTime: 300, threshold: 200, placeholder: "placeholder.jpg", onError: function(element) { console.log("Error loading " + element.data('src')); } }); }); </script>
Practical Use Case Example: Product Images for E-commerce
Lazy loading is particularly useful for e-commerce websites where many high-resolution product images can affect load time. Implementing lazy loading for product thumbnails allows for a faster browsing experience.
<div class="product-gallery"> <img class="lazy" data-src="product1.jpg" alt="Product 1" width="300" height="300"> <img class="lazy" data-src="product2.jpg" alt="Product 2" width="300" height="300"> <img class="lazy" data-src="product3.jpg" alt="Product 3" width="300" height="300"> </div>
Conclusion
Using jQuery for lazy loading images is a practical and effective way to enhance website performance. The jquery.lazy plugin provides an easy-to-implement solution for deferring image loads, helping to boost your site’s responsiveness, SEO, and user engagement.
By following this guide, you can implement lazy loading on your website quickly, ensuring that your visitors enjoy a fast and efficient experience even with multiple high-quality images on the page.