What is an Array
Nov 02, 24 Devgod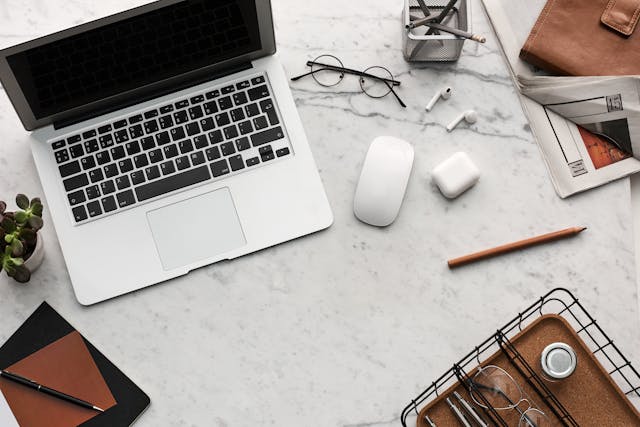
What is an Array?
In programming, an array is a data structure that holds a collection of elements, typically of the same type. Arrays are incredibly useful because they allow you to store multiple values in a single variable, making it easier to work with related data. Think of an array as a list, where each element has a specific position, or index, starting from zero. Arrays are foundational in programming and are used in almost every language, from JavaScript to Python, C++, and more.
Basic Syntax of an Array
In most programming languages, arrays are declared by specifying a name and the elements it contains. For example, in JavaScript, an array of numbers might look like this:
// Declaring an array of numbers
let numbers = [1, 2, 3, 4, 5];
Types of Arrays
Arrays can come in various types depending on the language and how they are used. Some of the most common types include:
1. Single-Dimensional Arrays
A single-dimensional array is the simplest type of array. It is a list of elements in a single row, where each element can be accessed by a single index. Single-dimensional arrays are often referred to as “linear arrays.”
// Example of a single-dimensional array in JavaScript
let fruits = ["Apple", "Banana", "Cherry"];
In this example, fruits[0]
will return "Apple", fruits[1]
will return "Banana", and so on.
2. Multi-Dimensional Arrays
A multi-dimensional array is an array that contains other arrays as its elements. The most common type is a two-dimensional array, which can be thought of as an array of arrays, like a matrix or grid. Each element in a two-dimensional array requires two indices to access, one for the row and one for the column.
// Example of a two-dimensional array in JavaScript
let matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
Here, matrix[0][0]
returns 1, matrix[1][1]
returns 5, and so forth.
3. Jagged Arrays
A jagged array is a type of multi-dimensional array where each row can have a different number of elements. Unlike standard multi-dimensional arrays, jagged arrays are not a fixed matrix. They are particularly useful in scenarios where data is irregularly shaped.
// Example of a jagged array in JavaScript
let jaggedArray = [
[1, 2],
[3, 4, 5],
[6]
];
Array Modes
Array modes refer to the various ways arrays can be used or the types of operations you can perform on them. Here are some of the most common array modes:
1. Traversing an Array
Traversing is the process of accessing each element in the array sequentially. Traversing an array allows you to perform actions on each element, such as printing them, modifying them, or calculating a total sum.
// Example of traversing an array in JavaScript
let numbers = [10, 20, 30, 40, 50];
for (let i = 0; i < numbers.length; i++) {
console.log(numbers[i]);
}
2. Searching in an Array
Searching involves finding a specific element within an array. This can be done in different ways depending on the programming language. In JavaScript, for instance, the includes()
method checks if an element exists within an array, and the indexOf()
method returns the index of the element.
// Searching for an element in an array
let fruits = ["Apple", "Banana", "Cherry"];
let index = fruits.indexOf("Banana"); // Returns 1
let exists = fruits.includes("Cherry"); // Returns true
3. Sorting an Array
Sorting arranges the elements in a specific order, either ascending or descending. Sorting is crucial for organizing data for faster searching, retrieval, or display. In JavaScript, the sort()
method can sort an array of strings alphabetically or numbers in ascending order.
// Sorting an array in JavaScript
let numbers = [50, 10, 40, 20, 30];
numbers.sort((a, b) => a - b); // Sorts in ascending order
4. Adding and Removing Elements
Arrays support methods for adding or removing elements. For instance, the push()
method adds an element to the end of an array, and pop()
removes the last element. Similarly, shift()
removes the first element, and unshift()
adds one or more elements to the beginning.
// Adding and removing elements in an array
let fruits = ["Apple", "Banana"];
fruits.push("Cherry"); // Adds Cherry to the end
fruits.pop(); // Removes the last element (Cherry)
5. Mapping and Filtering Arrays
The map()
and filter()
methods allow you to create new arrays based on certain conditions or transformations. map()
transforms each element in the array and returns a new array, while filter()
creates a new array containing elements that meet a specified condition.
// Mapping and filtering an array in JavaScript
let numbers = [1, 2, 3, 4, 5];
let doubled = numbers.map(num => num * 2); // Doubles each element
let even = numbers.filter(num => num % 2 === 0); // Filters even numbers
Conclusion
Arrays are fundamental structures that enable efficient data handling in programming. Understanding the different types of arrays—single-dimensional, multi-dimensional, and jagged arrays—along with the modes of usage like traversing, searching, sorting, adding/removing, and mapping/filtering, is crucial for any programmer. Arrays enhance data management, making it easier to work with lists, tables, and other forms of structured information. By mastering arrays, you can develop a solid foundation for tackling more complex data structures and algorithms in your programming journey.